Python Operator Precedence: Python, a versatile and widely used programming language, offers a rich set of operators for performing various operations on data. To harness the full power of Python, it is crucial to comprehend the concept of operator precedence. Operator precedence determines the order in which operations are executed within an expression. This article delves into the intricacies of Python operator precedence, elucidating its importance and providing practical examples to enhance your understanding.
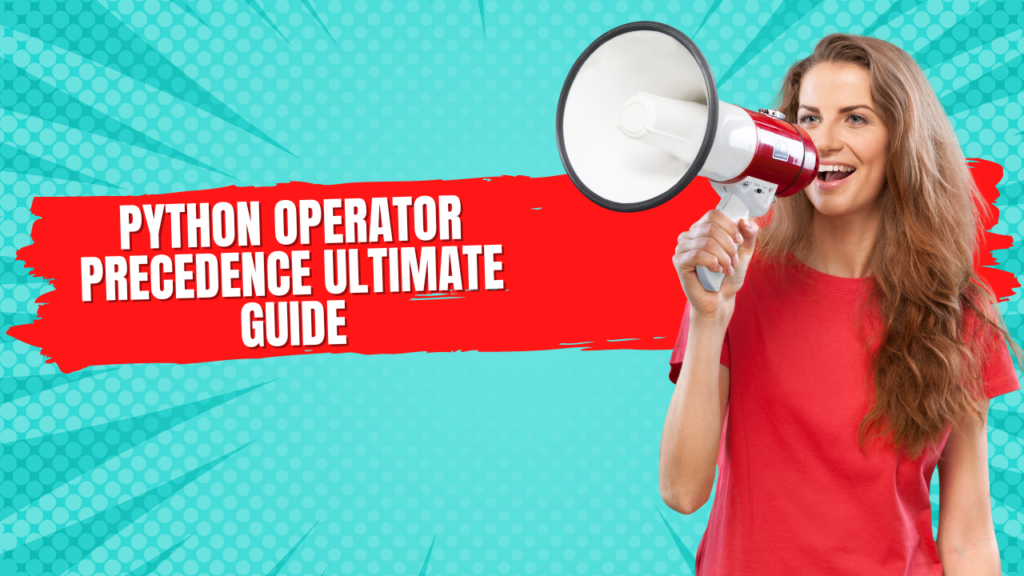
Python Operator Precedence: The Basics of Operator Precedence:
Operator precedence refers to the rules that dictate the order in which operators are evaluated in an expression. In Python, like in many programming languages, certain operators take precedence over others, ensuring that expressions are evaluated predictably. For instance, multiplication has higher precedence than addition, so in the expression 2 + 3 * 4
, the multiplication operation is performed first, resulting in 14.
Python Operator Precedence – Understanding the Hierarchy
Python operators can be categorized into various groups based on their precedence levels. The hierarchy from highest to lowest precedence is as follows:
- Parentheses: Parentheses have the highest precedence and are used to override the default precedence. Expressions within parentheses are evaluated first.
- Exponentiation: The exponentiation operator (
**
) has the next highest precedence. It is used to raise a number to a power.
- Multiplication, Division, and Floor Division: Multiplication (
*
), division (/
), and floor division (//
) have the same precedence level and are evaluated from left to right.
- Addition and Subtraction: Addition (
+
) and subtraction (-
) also share the same precedence level and are evaluated from left to right.
- Bitwise Shifts: Bitwise left shift (
<<
) and bitwise right shift (>>
) come next in precedence.
- Bitwise AND: Bitwise AND (
&
) is evaluated after bitwise shifts.
- Bitwise XOR and OR: Bitwise XOR (
^
) and bitwise OR (|
) have the same precedence level and are evaluated from left to right.
- Comparison Operators: Comparison operators (e.g.,
<
,<=
,>
,>=
) are evaluated next, and they return Boolean values. - Equality Operators: Equality operators (
==
and!=
) have lower precedence than comparison operators. - Logical AND: Logical AND (
and
) is used for boolean operations and has lower precedence than equality operators. - Logical OR: Logical OR (
or
) is the lowest precedence among the logical operators.
Python Operator Precedence: Understanding these precedence levels is crucial for writing correct and efficient Python code.
Let’s explore a few practical examples to illustrate the significance of operator precedence.
Example 1:
result = 10 + 2 * 3 print(result)
In this example, multiplication takes precedence over addition. The result will be 16, as the multiplication operation is evaluated first.
Example 2:
result = (10 + 2) * 3 print(result)
By using parentheses, we override the default precedence. The result will be 36, as the addition operation inside the parentheses is evaluated first.
Example 3:
result = 2 + 3 ** 2 print(result)
Exponentiation has higher precedence than addition. The result will be 11, as the exponentiation operation is performed first.
In the dynamic world of Python programming, unlocking the secrets of operator precedence isn’t just a skill – it’s the ultimate game-changer for crafting code that stands out from the crowd. Picture this: you, armed with the knowledge of operator hierarchy, effortlessly orchestrating expressions in perfect harmony, ensuring your code dances to the intended rhythm. The stakes get higher as projects grow more intricate, making your mastery of this skill a badge of honor among developers.
But here’s the real scoop: it’s not just about understanding; it’s about conquering the coding jungle with finesse! The prowess to navigate through the labyrinth of operators, especially in those complex coding terrains, becomes your secret weapon. It’s the key to not just writing code but orchestrating a symphony of logic where every note plays in flawless succession.
Now, here’s the kicker: Want to ascend to Python programming greatness? Dive headfirst into the playground of operators and parentheses, experimenting with a myriad of combinations. It’s not just a learning exercise; it’s a thrill ride that transforms your understanding of operator precedence into an intuitive superpower.
Think of it as a Jedi mastering the lightsaber – your code becomes an elegant dance, a spectacle of efficiency and precision. And the best part? It’s not just theory; it’s a practical journey that transforms you from a Python enthusiast to a programming maestro.
So, why settle for ordinary coding when you can command the Python stage like a virtuoso? Unleash the full potential of your programming prowess – understand, experiment, and transcend the ordinary with Python operator precedence. Your code will thank you, and your programming journey will never be the same again!